OpenCV+Python+Webカメラ リアルタイム特徴量検出
- Takeshi Suetani
- 2018年4月30日
- 読了時間: 2分
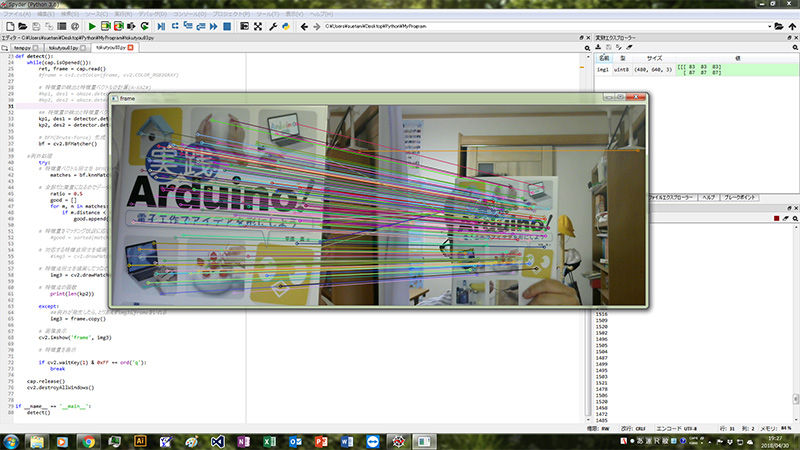
とりあえず成功。
・検出方法をA-KAZEからSURFに変更
・検出できないときのための例外処理を追加
(試行錯誤の跡がコメントに存在します)
///////////以下、Pythonのコード(要Webカメラ)
import cv2 import numpy as np # 比較元の画像 img1 = cv2.imread("img/image.png") #img1 = cv2.cvtColor(cv2.imread("img/image.png"),cv2.COLOR_RGB2GRAY)
# A-KAZE検出器の生成 #akaze = cv2.AKAZE_create()
#SURF検出器の生成 ()内の数値でヘッセの閾値を設定できる detector = cv2.xfeatures2d.SURF_create()
# カメラ cap = cv2.VideoCapture(0) cap.set(3, 640) cap.set(4, 480) cap.set(5, 15) # detect def detect(): while(cap.isOpened()): ret, frame = cap.read() #frame = cv2.cvtColor(frame, cv2.COLOR_RGB2GRAY) # 特徴量の検出と特徴量ベクトルの計算(A-KAZ#) #kp1, des1 = akaze.detectAndCompute(img1, None) #kp2, des2 = akaze.detectAndCompute(frame, None) ## 特徴量の検出と特徴量ベクトルの計算(SURF) kp1, des1 = detector.detectAndCompute(img1, None) kp2, des2 = detector.detectAndCompute(frame, None) # BFM(Brute-Force) 生成 bf = cv2.BFMatcher() #例外処理 try: # 特徴量ベクトル同士を BFM(Brute-Force)&KNN でマッチング matches = bf.knnMatch(des1, des2, k=2) # 特徴量データを間引き
ratio = 0.5 good = [] for m, n in matches: if m.distance < ratio * n.distance: good.append([m]) # 特徴量をマッチング状況に応じてソートする #good = sorted(matches, key = lambda x : x[1].distance)
# 対応する特徴点同士を描画 #img3 = cv2.drawMatchesKnn(img1, kp1, frame, kp2, good[:30], None, flags=2) # 特徴点同士を描画してつなぐ img3 = cv2.drawMatchesKnn(img1, kp1, frame, kp2, good, None, flags=2) # 特徴点の個数 print(len(kp2)) except: ##例外が発生したら、とりあえずimg3にframeをいれる img3 = frame.copy() # 画像表示 cv2.imshow('frame', img3) # 特徴量を表示 if cv2.waitKey(1) & 0xFF == ord('q'): break cap.release() cv2.destroyAllWindows() if __name__ == '__main__': detect()
コメント